1. Singleton Pattern Meaning
- Singleton: A design pattern for constructing a class which limits construction to a single instance.
- This single instance is contained within the class itself.
- Typically this class is then exposed globally.
- Advantages:
- Globally accessible instance
- Ensures only a single instance is ever created.
- Disadvantages:
- Globally accessible instance: anyone can use it anytime, so no control over where it would be used.
- Only initializes on first access.
- Not thread safe in all situation (safe using VS2015+)
2. Rules:
- The constructor be made hidden. (private)
- Static instance accessor function
- Doesn't need to be named 'GetInstance()' but that's the standard way - A private static instance within the class
- A global pointer to access the static instance
3. Codes
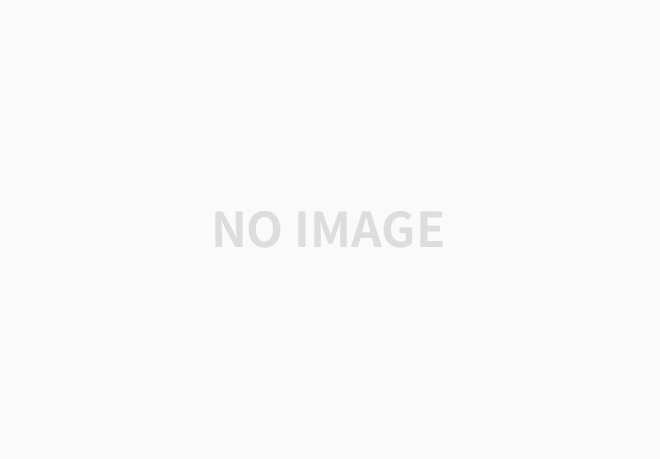
- Declare a constructor and destructor in private section. And make a static member pointer variable.
- Define a static function 'GetInstance()' inside of the header file, not .cpp file. Most of cases, if the definition is more than 2 lines, it should be defined in cpp file, but in this case, it is possible to define it in the header file, as an exception.
- And with the keyword 'new', assign the NetworkManager pointer to the static member pointer variable.
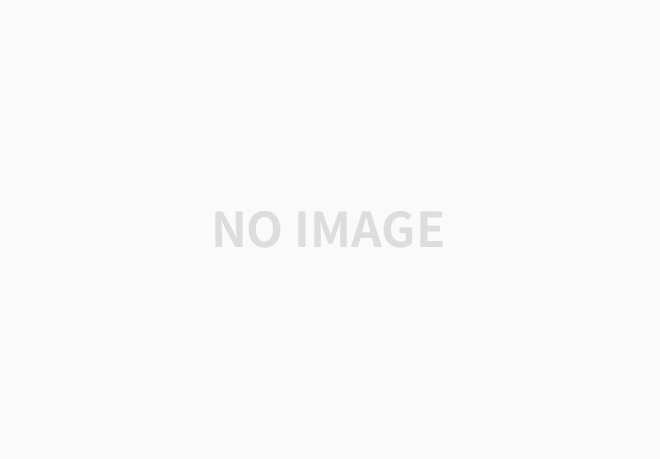
- In order to initialize the static member pointer variable, you'd better initialize in the .cpp file, the outside of the header file. About singleton, the constructor is in the private section, so it can't make an instance in the main.cpp, so it creates a pointer instance with static.
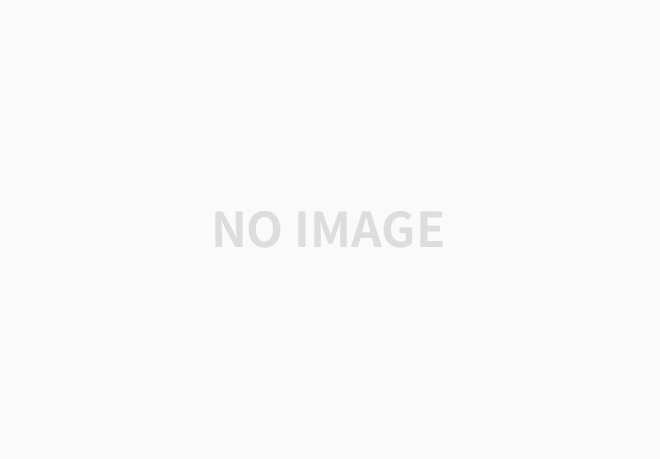
- In the main.cpp, with 'getInstance()', you can assign the NetworkManager singleton instance.
4. Singletons in Games
- Singletons are sometimes used to manage systems of games and game engines which need to be frequently used throughout many other sections of code.
- Often used in:
- Audiomanagers
- InputManagers
- Debuggers / loggers
- Some architectures restric to only a single Main or Game singleton which contains a single static instance of all other classes that a game may need accessible.
- Other Architectures prefer as restrictive of a system as possible to eliminate accidental use and abuse of systems.
'Programming > Programming' 카테고리의 다른 글
[GAME311] Lab1 Review (1) | 2023.10.17 |
---|---|
[GAME300] Graphic Programming (0) | 2023.09.19 |
[GAME300] Graphic Programming 1~2 (0) | 2023.09.15 |
[C++] 구조체에서의 static 멤버 (0) | 2023.09.09 |